Ahrengot last won the day on
Ahrengot had the most liked content!
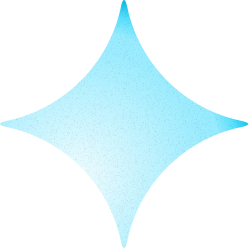
Ahrengot
Business-
Posts
60 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Store
Blog
Product
Showcase
FAQ
Downloads
Everything posted by Ahrengot
-
Can i use delayedCall() to change a variable or a setter?
Ahrengot replied to Ahrengot's topic in GSAP (Flash)
interesting ... set up a little test: public class Main extends Sprite { public var myVariable:Boolean = false; private var _var:Boolean = false; public function Main() { addEventListener(Event.ADDED_TO_STAGE, init, false, 0, true) } private function init(e:Event):void { stage.addEventListener(MouseEvent.CLICK, onClick); } private function onClick(e:MouseEvent):void { // TweenMax.to(this, 0, {variable:!_var, delay:1}); // TweenMax.to(this, 0, {delay:1, onComplete:variable, onCompleteParams:[!_var]}); // TweenMax.to(this, 0, {myVariable:!myVariable, delay:1, onComplete:trace, onCompleteParams:[string(this + " myVariable: ") + myVariable]}); } public function set variable($value:Boolean):void { _var = $value; trace(this + " variable changed to: " + _var); } } With the public variable it works fine: TweenMax.to(this, 0, {myVariable:!myVariable, delay:1, onComplete:trace, onCompleteParams:[string(this + " myVariable: ") + myVariable]}); With the public setter it fails Test 1: TweenMax.to(this, 0, {variable:!_var, delay:1}); throws: ReferenceError: Error #1077: Illegal read of write-only property variable on Main. at com.greensock::TweenLite/init() at com.greensock::TweenMax/init() at com.greensock::TweenMax/renderTime() at com.greensock.core::SimpleTimeline/renderTime() at com.greensock::TweenLite$/updateAll() Test 2: TweenMax.to(this, 0, {delay:1, onComplete:variable, onCompleteParams:[!_var]}); throws: 1120: Access of undefined property variable. So it seems that ActionScript doesn't completely treat setter methods as public properties, and if i want to do a timed call to a setter i need the function inbetween to modify the setter. -
Hey tweening rock stars! Quick question: Is there any way to define variables or setter methods with delayedCall() or any of the callbacks in TweenMax/Lite? I mean without creating a function inbetween like this: TweenMax.delayedCall(1, setVar, [value]); function setVar($value:*):void { myVar = $value; }
-
Ha! Turned out to be somewhere else in my code i'd messed up. I use a state setter/getter as an alternative way of toggling play/pause/stop on my player, and whenever the video was ready to start playback i manually set the state to be paused so that the state getter returns an accurate value, i just didn't keep in mind they won't always have autoplay disabled. Simply needed to change this private function onNetstatus(e:NetStatusEvent):void { if (e.info.code == "NetStream.Play.Start" || _loader.bufferProgress == 1) { _loader.netStream.removeEventListener(NetStatusEvent.NET_STATUS, onNetstatus); state = VideoState.PAUSED; dispatchEvent(new VideoPlayerEvent(VideoPlayerEvent.READY)); } } to this: private function onNetstatus(e:NetStatusEvent):void { if (e.info.code == "NetStream.Play.Start" || _loader.bufferProgress == 1) { _loader.netStream.removeEventListener(NetStatusEvent.NET_STATUS, onNetstatus); vars.autoPlay? state = VideoState.PLAYING : state = VideoState.PAUSED; dispatchEvent(new VideoPlayerEvent(VideoPlayerEvent.READY)); } } Sorry 'bout that - I feel so silly
-
Hey Jack! I'm working on this player http://www.ahrengot.com/playground/circular-scrubbing - It's my custom Video Player (Talking about the Actual Player, not the graphic interface here) built on top of your VideoLoader class with some extended features, getters, events and implemented under my own IVideoPlayer Interface class. It was a real joy to build, because the fundament was so solid, but i discovered something that made me scratch my head: If you set autoPlay:true on a VideoLoader it will dispatch a pause event before it starts playing. Is that how it's supposed to be? I could pop the hood on your VideoLoader and try to followed every single function call from instantiation to playback starts, but chances are i'd miss something. I'm really just looking for the reason why it works this way.
-
It amazes me how fast you are at fixing and updating things like this. Many thanks
-
Hey Jack! I am working on another video player that implements your awesome VideoLoader, and i noticed some strange behavior. Since you added the fitWidth and fitHeight setters, whenever you call them and thereby call the _update() method in ContentDisplay a new background is drawn in the ContentDisplay on top of the old one instead of replacing it. This means Content Display will report a wrong width/height, dispatch mouse events it shouldn't etc. as the background expands beyond the limits provided by the new width/height. ContentDisplay.as line 110 if (_fitWidth > 0 && _fitHeight > 0) { graphics.beginFill(_bgColor, _bgAlpha); graphics.drawRect(left, top, _fitWidth, _fitHeight); graphics.endFill(); } This fixed it for me: if (_fitWidth > 0 && _fitHeight > 0) { graphics.clear(); graphics.beginFill(_bgColor, _bgAlpha); graphics.drawRect(left, top, _fitWidth, _fitHeight); graphics.endFill(); }
-
Finding out where AutoFitArea moves your objects
Ahrengot posted a topic in TransformManager (Flash)
Hey! I'm utilizing AutoFitArea in one of my projects, but haven't had a lot of experience working with that particular class yet, and i'm a little confused as to how it works. I've setup the area like this: _autoFitArea = new AutoFitArea(this, 0, 0, stage.stageWidth, stage.stageHeight); _autoFitArea.attach(_background, "proportionalOutside", "center", "center"); added a stage.RESIZE listener and in that handler method i do this: private function onStageResize(e:Event = null):void { _autoFitArea.width = stage.stageWidth; _autoFitArea.height = stage.stageHeight; } Everything works as it should, but at some point later in the code i need to do a bitmap.draw() of the _background DisplayObject. And to do that accurately i need the position of _background, but when i read the x and y properties of _background it reports back 0. Reading x/y properties from the AutoFitArea itself reports 0 back as well. Is there any way to get those values, and how come they return 0? Is that how it's supposed to work? -
How to know if a VideoLoader's video is ready
Ahrengot replied to Ahrengot's topic in Loading (Flash)
When i say ready i mean that the video has buffered enough to start playback. Preferably buffered the amount described by the bufferTime property. I tried the BUFFER_FULL event, but that doesn't seem to dispatch when autoPlay is set to false. I created a little example to show what i mean. (note i add a CLICK listener after onInit is fired, that makes the video play/pause whenever the stage is clicked, to show that BUFFER_FULL is only dispatched after play is called the first time) package { import com.greensock.events.LoaderEvent; import com.greensock.loading.VideoLoader; import flash.display.Sprite; import flash.events.*; public class Main extends Sprite { private var _loader:VideoLoader; public function Main() { if (stage) init(); addEventListener(Event.ADDED_TO_STAGE, init, false, 0, true); } private function init(e:Event = null):void { removeEventListener(Event.ADDED_TO_STAGE, init); loadVideo(); } private function loadVideo():void { _loader = new VideoLoader("assets/video/surfer.mp4", { container:this, autoPlay:false, onInit:onMetaData }); _loader.addEventListener(VideoLoader.VIDEO_BUFFER_FULL, onEventRecieved); _loader.load(); } private function onMetaData(e:Event):void { stage.addEventListener(MouseEvent.CLICK, onClickStage); buttonMode = true; } private function onClickStage(e:MouseEvent):void { (_loader.videoPaused)? _loader.playVideo() : _loader.pauseVideo(); } private function onEventRecieved(e:Event):void { trace(this + " Video fully buffered!"); } } } Here's my example: -
Hey! I was wondering what the best way to know if my VideoLoader's video is ready for playback when autoPlay is set to false. Right now i'm listening to NetStatusEvent's from the VideoLoader's netstream like so: var loader:VideoLoader = new VideoLoader(url, {autoPlay:false}); loader.load(); loader.netStream.addEventListener(NetStatusEvent.NET_STATUS, onNetstatus); function onNetstatus(e:NetStatusEvent):void { if (e.info.code == "NetStream.Play.Start" || loader.bufferProgress == 1) { loader.netStream.removeEventListener(NetStatusEvent.NET_STATUS, onNetstatus); dispatchEvent(new VideoPlayerEvent(VideoPlayerEvent.READY)); } } note: VideoPlayerEvent is my own event class i'm using for various video players. It's working as it should, but i'm wondering if i'm prone to any errors doing it this way or if any of your LoaderEvent's does the same thing. Otherwise, would it be possible to have you add a READY event that get's dispatched whenever a video is ready for playback (much like YouTube does through it's API).
-
Hello Jack, I was wondering if it would be possible for you to add a feature, where one could easily define easing for a tween by combining different easing methods for in and out. I often find my self wanting to combine 2 pre-excisting ease methods for in and out, like Expo.easeIn and Back.easeOut. I realize you made the custom ease builder where i could create a curve representing what i'm looking for, copy the array it outputs and create my custom ease function that way, but it takes a long time to perfectly match the pre-excisting easing functions or create something that looks as good as they do. Of course the CustomEase builder is fantastic if you're trying to create something completely unique, but most of the time i'm looking to just combine 2 ease methods. Would this be possible at all?
-
You can use timeScale on individual tweens or globalTimeScale on TweenMax. If you're using TimelineMax it also has a timeScale. You could also manipulate the totalProgress property on TweenMax/TimelineMax, which works like the scrubber on a 'real' timeline. timeScale: http://www.greensock.com/as/docs/tween/ ... #timeScale globalTimeScale: http://www.greensock.com/as/docs/tween/ ... lTimeScale totalProgress: http://www.greensock.com/as/docs/tween/ /edit: .. bah, i was too slow
-
You've misspelled TweenLite. Change you code to this import com.greensock.TweenLite; import com.greensock.TimelineMax; import com.greensock.easing.*;
-
Sorry, misunderstood your question and haven't found the delete post button on this forum yet
-
Great site! very nice work
-
It's because you aren't rounding off your tween properties, and text needs to be on whole pixels, so it jumps/snaps back and forth between pixels. this fixes it: var timeline:TimelineLite = new TimelineMax({onComplete:myFunction}); timeline.append (TweenMax.from(mc1,5,{blurFilter:{blurX:20},x:0,ease:Strong.easeOut,alpha:0, roundProps:["x"]})); timeline.append (TweenMax.from(mc2,6,{blurFilter:{blurX:20},x:0,ease:Strong.easeOut,alpha:0, roundProps:["x"]})); timeline.append (TweenMax.from(mc3,7,{blurFilter:{blurX:20},x:0,ease:Strong.easeOut,alpha:0, roundProps:["x"]}));
-
Yes, i am aware of those and that's why i figured i could use TweenLite in my panning class instead of writing my own tweening/easing code. btw, i like the warning massage that appears when you switch to Adobe's tweening engine Thanks for answering my question so quickly.
-
It was a little hard to figure out what you wanted to do, but i think this should work. var segment01_TL:TimelineMax = new TimelineMax({paused:false}); var cycles:uint = 3; var spinDuration:Number = 1; var tags:Array = [tag1container, tag2container, tag3container, tag4container]; var stagger:Number = 0; for (var i:int = 0; i < tags.length; i++) { var timeline:TimelineLite = new TimelineLite(); var vars:Object = { transformMatrix: { skewY: -180 }, paused:true, repeat:cycles * 1 + 1, yoyo:true, ease:Linear.easeNone }; timeline.insertMultiple([TweenMax.from(tags[i].["tag" + int(i + 1)], spinDuration, vars), TweenMax.from(tags[i], 1, { autoAlpha:0 } ) ] ); segment01_TL.append(timeline, stagger); stagger -= 3.15; }
-
Well, both yes and no. I'm not asking you to run through my entire class and optimize everything it it. I don't believe that's what this forum is about I am asking if there's any possible complications in my pan() method, where i'm doing potentially 30 tween overwrites per second(default framerate), if it runs for minutes on end doing thousands of overwrites and new tween instantiations. I've, as you metioned, set the overwriting mode to ALL_IMMEDIATE as that's supposed to be the best option performance wise and made sure to kill tweens where relevant. I've tested it out and found nothing to go wrong so far. Just wanted to make sure. The reason I posted the entire class is so people here can just copy-paste it into their own projects if they'd like to use it
-
Hello tween masters! I've built an image panner class (You know, the effect where you pan around a large image inside a smaller area, like the collection browser on this site: http://www.minus.dk/). Everything is working and running really smoothly (test link: http://www.ahrengot.com/playground/panning/) and as you can see it adapts dynamically when you resize. I'm using TweenLite to do the tweening as i'm thinking of expanding the functionality later by adjusting the Vars object of TweenLite. My question is, is there anything i should be aware of when i'm doing a lot of tweening overrides like i am here? This is the tweening mechanism (look in pan()): /** * Start panning */ public function start():void { _target.stage.addEventListener(MouseEvent.MOUSE_MOVE, pan); } private function pan(e:MouseEvent = null):void { // Horizontal panning mechanism (_panX)? _targetX = getXTarget() : _targetX = _target.x; // Vertical panning mechanism (_panY)? _targetY = getYTarget() : _targetY = _target.y; // Tween TweenLite.to(_target, _ease, { y:_targetY, x:_targetX, overwrite:OverwriteManager.ALL_IMMEDIATE } ); } private function getXTarget():int { if (_target.stage.mouseX >= _minX && _target.stage.mouseX <= _maxX) { _posX = _target.stage.mouseX - _minX; } if (_target.stage.mouseX > _maxX) _posX = _maxX - _minX; else if (_target.stage.mouseX < _minX) _posX = 0; _posXFactor = (_posX) / _measureDistX; return int(0 - ((_target.width - _area.width) * _posXFactor)); } private function getYTarget():int { if (_target.stage.mouseY >= _minY && _target.stage.mouseY <= _maxY) { _posY = _target.stage.mouseY - _minY; } if (_target.stage.mouseY > _maxY) _posY = _maxY - _minY; else if (_target.stage.mouseY < _minY) _posY = 0; _posYFactor = (_posY) / _measureDistY; return int(0 - ((_target.height - _area.height) * _posYFactor)); } Here's the entire class if anyone would like to use it package ahrengot.ui { import com.greensock.OverwriteManager; import com.greensock.TweenLite; import flash.events.Event; import flash.events.MouseEvent; import flash.display.DisplayObject; import flash.geom.Rectangle; public class PanArea { private var _target:DisplayObject; private var _area:Rectangle; // Panning properties private var _panY:Boolean; private var _panX:Boolean; private var _minY:Number; private var _maxY:Number; private var _minX:Number; private var _maxX:Number; private var _ease:Number; // Dynamic properties private var _posXFactor:Number; private var _measureDistX:Number; private var _posX:Number; private var _posYFactor:Number; private var _measureDistY:Number; private var _posY:Number; private var _targetX:Number; private var _targetY:Number; /** * Pans a DisplayObject within a specified area. Optionally applies a pan margin * @param $panTarget - Target to pan * @param $panArea - Area to pan within. Can be a Stage, a DisplayObject or a rectangle * @param $startImmidiately - Whether or not to start the panning immidiately. (You start this manually by calling the start() method) * @param $horizontalPanning - Pan horizontal? * @param $verticalPanning - Pan vertically? * @param $ease - Ease (duration property sent to TweenLite - Value is in seconds) * @param $marginTop - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginBottom - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginLeft - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginRight - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. */ public function PanArea($panTarget:DisplayObject, $panArea:*, $startImmidiately:Boolean = true, $horizontalPanning:Boolean = true, $verticalPanning:Boolean = true, $ease:Number = 0.35, $marginTop:Number = 0, $marginBottom:Number = 0, $marginLeft:Number = 0, $marginRight:Number = 0) { _target = $panTarget; ($panArea is Rectangle)? _area = $panArea : _area = new Rectangle($panArea.x, $panArea.y, $panArea.width, $panArea.height); _panY = $horizontalPanning; _panX = $verticalPanning; _minY = $marginTop; _maxY = _area.height - $marginBottom; _minX = $marginLeft; _maxX = _area.width - $marginRight; _measureDistX = _maxX - _minX; _measureDistY = _maxY - _minY; _ease = $ease; if ($startImmidiately) start(); } private function pan(e:MouseEvent = null):void { // Horizontal panning mechanism (_panX)? _targetX = getXTarget() : _targetX = _target.x; // Vertical panning mechanism (_panY)? _targetY = getYTarget() : _targetY = _target.y; // Tween TweenLite.to(_target, _ease, { y:_targetY, x:_targetX, overwrite:OverwriteManager.ALL_IMMEDIATE } ); } private function getXTarget():int { if (_target.stage.mouseX >= _minX && _target.stage.mouseX <= _maxX) { _posX = _target.stage.mouseX - _minX; } if (_target.stage.mouseX > _maxX) _posX = _maxX - _minX; else if (_target.stage.mouseX < _minX) _posX = 0; _posXFactor = (_posX) / _measureDistX; return int(0 - ((_target.width - _area.width) * _posXFactor)); } private function getYTarget():int { if (_target.stage.mouseY >= _minY && _target.stage.mouseY <= _maxY) { _posY = _target.stage.mouseY - _minY; } if (_target.stage.mouseY > _maxY) _posY = _maxY - _minY; else if (_target.stage.mouseY < _minY) _posY = 0; _posYFactor = (_posY) / _measureDistY; return int(0 - ((_target.height - _area.height) * _posYFactor)); } ////////////////////// PUBLIC METHODS ////////////////////// /** * Start panning */ public function start():void { _target.stage.addEventListener(MouseEvent.MOUSE_MOVE, pan); } /** * Stop panning */ public function stop($killActiveTweens:Boolean = true):void { if ($killActiveTweens) TweenLite.killTweensOf(_target); _target.stage.removeEventListener(MouseEvent.MOUSE_MOVE, pan); } /** * Sets the area in which the panning is calculated. * @param $panArea * @param $marginTop - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginBottom - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginLeft - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. * @param $marginRight - Defines a margin area where panning isn't registered. ie. of you use a top and bottom margin of 100px within an area 400px high, the active scroll area will be 200px high. */ public function adjustPanArea($panArea:*, $marginTop:Number = 0, $marginBottom:Number = 0, $marginLeft:Number = 0, $marginRight:Number = 0) { stop(); _area = null; ($panArea is Rectangle)? _area = $panArea : _area = new Rectangle($panArea.x, $panArea.y, $panArea.width, $panArea.height); _minY = $marginTop; _maxY = _area.height - $marginBottom; _minX = $marginLeft; _maxX = _area.width - $marginRight; _measureDistX = _maxX - _minX; _measureDistY = _maxY - _minY; _target.x = getXTarget(); _target.y = getYTarget(); start(); } /** * Remove listeners and readies for Garbage Colelction */ public function destroy():void { stop(); _target = null; _area = null; } } }
-
Tween effect only works the first time properly
Ahrengot replied to mrlem313's topic in GSAP (Flash)
Post some code, so we can see what's going on. -
I haven't really ever had to do what you're trying to accomplish here, but putting your button in a sprite and animating tint on the wrapper sprite should work.
-
Declaring TweenMax Objects and use them later
Ahrengot replied to MultipleParadox's topic in GSAP (Flash)
sure... constructor() { var mainTimeline:TimelineLite = new TimelineLite(); mainTimeline.pause(); var anim1:TimelineLite = setupAnim1(); var anim2:TimelineLite = setupAnim2(); } I'd really recommend reading through the ASDOC's as you actually have an incredible amount of control over the progress of a timeline. You can even use TweenLite, TweenMax to tween a timeline's overall progress (thereby easing the progress among other cool things). -
Ha! thanks for that I used to draw bitmap proxies, hide the original, manipulate those proxies, remove them and unhide original agian when i was done with he 3D transformations.
-
Yes, that seems like the best way to go. Thanks a lot
-
Ahh yeah, it makes sense in the context where you're just unloading the current content to load some other content, but what confuses me, it is still left behind after you call both the unload() and dispose() method. What i'm looking for is a way to remove EVERYTHING on the loader classes when i'm done using them. would there be any reason not to remove ContentDisplay on unload() and add it again if the user calls load()? It seems like you've already thought out almost every possible scenario, so i'm just trying to learn how to best use these awesome classes