React and GSAP timelines callbacks
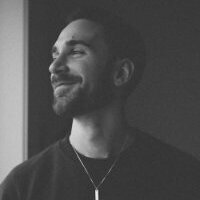
By
Daniel Hult
in GSAP
Moderator Tag
-
Recently Browsing 0 members
- No registered users viewing this page.
By
Daniel Hult
in GSAP
Recommended Posts
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now