Loading shared assets
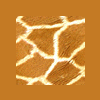
By
friendlygiraffe
in Loading (Flash)
Moderator Tag
-
Recently Browsing 0 members
- No registered users viewing this page.
By
friendlygiraffe
in Loading (Flash)
Recommended Posts
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now