Mapping through arrays - list items the react way, with conditional logic
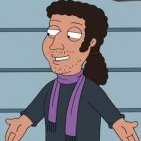
By
UnioninDesign
in GSAP
Moderator Tag
Warning: Please note
This thread was started before GSAP 3 was released. Some information, especially the syntax, may be out of date for GSAP 3. Please see the GSAP 3 migration guide and release notes for more information about how to update the code to GSAP 3's syntax.
-
Recently Browsing 0 members
- No registered users viewing this page.
Recommended Posts
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now